Analytics
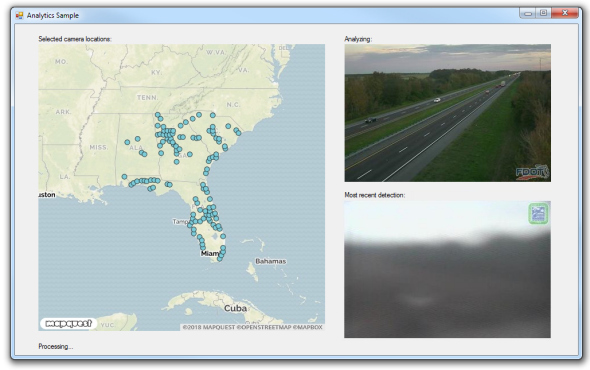
Vizzion's Analytics sample application illustrates a number of considerations when developing an application that performs analytics on camera images, including decluttering camera data, refreshing camera data, refreshing camera images, camera service load balancing, and on-vehicle cameras.
As a simple example, this sample application will detect any rapid changes in brightness in camera images, e.g., due to lighting turning on or off, a malfunctioning camera, or even a lightning strike.
In addition to pseudocode illustrating the recommended techniques, the full sample application source code is available for download.
Decluttering Camera Data
Many cameras in Vizzion’s traffic camera database are located at close or identical locations to other cameras. However, for some analytics applications, it may not be necessary to process images from every camera image to achieve accurate results. By establishing an evenly spaced grid of locations, and by selecting a single camera from each grid location, processing time, resources and service costs can be reduced substantially.
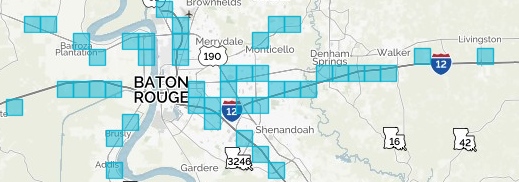
This pseudocode illustrates how to first retrieve all cameras in a grid area using GetCamerasInBox, and then select a camera for each grid location based on service status, viewpoint, or other criteria:
Pseudocode |
---|
GridTop = 50, GridLeft = -100, GridBottom = 45, GridRight = -50 CamerasDataSet = GetCamerasInBox2(GridLeft, GridRight, GridBottom, GridTop, "", 0, <password>) CameraList = empty list For (Longitude = GridLeft to GridRight in steps of 0.1) For (Latitude = GridBottom to GridTop in steps of 0.1) For Each Camera in CamerasDataSet If Camera is near Latitude/Longitude, and Camera is in service Add Camera to CameraList |
Refreshing Camera Data
Camera data in Vizzion's traffic camera database updates at regular intervals. To ensure camera data stays up-to-date, analytics applications should automatically refresh camera data at least every 15 minutes. In this sample application, refreshing camera data also means recalculating the grid of cameras to be processed.
Refreshing Camera Images
Camera images in Vizzion's traffic camera database update at a regular interval, varying from half a second to one hour. This interval, in milliseconds, is stored in the UpdateFrequency field for each camera (see GetCamerasInBox). Analytics applications should process images from cameras at the update frequency of the camera, or slower if frequent processing is not required.
Camera Service Load Balancing
Analytics applications should attempt to spread processing evenly over the desired cycle frequency, rather than processing all cameras as fast as possible and then idling for the remainder of the cycle time. Analytics applications should also attempt to spread processing evenly over all regions, rather than processing all cameras in one region before moving on to the next.
In addition to helping Vizzion and its providers make the best of use of server resources, these techniques also minimize the processing resources required by the analytics application.
This pseudocode illustrates one method to accomplish load balancing, which is to allocate one processing thread to each camera state (or region, country, or continent, depending on the number of threads required), and to introduce a delay in between each camera:
Pseudocode |
---|
Regions = GetRegions(<password>) For Each State in Regions Create a thread to process the State: TargetCycleTime = 1 minute StartTime = Now For Each Camera in CameraList If Camera is not part of State, go to next Camera Image = GetCameraImage(Camera.CameraID, 360, 240, 8, 0, <password>).Image Process Image Recalculate AverageProcessingTime CycleTimeElapsed = Now - StartTime CycleTimeRemaining = TargetCycleTime - CycleTimeElapsed Wait (CycleTimeRemaining / CamerasRemaining) - AverageProcessingTime |
Note: If using HttpClient, set UseCookies to false to avoid all traffic going to a single server.
On-Vehicle Cameras
Vizzion's traffic camera coverage includes both traditional fixed cameras and live imagery from on-vehicle cameras. Images from these on-vehicle cameras are submitted at fixed locations and headings in the road network, referred to as "hotspot cameras" (see Hotspot Cameras). Hotspot cameras can greatly increase the extent and density of coverage in some areas.

Example of 4 hotspot cameras at an interchange
While hotspot cameras appear very similar to traditional fixed cameras in the Camera API data, they can require special consideration in analytics applications. Since a particular hotspot camera image may be captured by any number of different on-vehicle cameras, and the capture location of the image may vary by up to 200m, there is less consistency between one image and the next. Hotspot cameras also tend to change service status more frequently than traditional fixed cameras.
In this sample application, on-vehicle cameras are used, but a larger brightness change is required for a detection due to the greater variation in images.
Note: Hotspot cameras can be identified by the presence of the Hotspot field in method responses.
Downloads
Download | Details |
---|---|
Source code | Requires Visual Studio 2012 or later |